Sure! Here’s an explanation of 10 Python methods that can significantly enhance the efficiency and readability of your code without diving into actual code examples.
Table of Contents

List Comprehensions:
In Python, list comprehensions provide a condensed method for creating lists. Compared to conventional loops, they provide a more succinct syntax and let you create lists based on preexisting lists. List comprehensions allow you to develop more understandable and efficient code by cutting down on the number of lines required for list operations.
Dictionary Comprehensions:
Dictionary comprehensions offer a succinct syntax for building dictionaries in Python, just like list comprehensions do. They let you create dictionaries with a concise, easily understood syntax, which improves the expressiveness and efficiency of your code while handling dictionary operations.
Generator Expressions:
In Python, generator expressions provide a memory-efficient method of iterating over lengthy data sequences. Generator expressions create values one at a time as needed, as contrast to list comprehensions, which build a whole list in memory. This can greatly lower memory use and enhance code performance, particularly when working with big datasets.

Map and Filter Functions:
Python’s map() and filter() functions provide strong methods for applying functions on iterables. Whereas filter() selectively removes items from an iterable according to a predicate function, map() applies a function to every item in an iterable. You can write more concise and expressive code for data transformation and filtering by utilizing these functions, which will also increase the readability and performance of your code.
Lambda Functions:
You can define tiny, inline functions in Python using lambda functions, sometimes referred to as anonymous functions. They are in very handy when you need to send an argument from one basic method to another, such in the case of filter() or map(). By removing the requirement to write a distinct named function for simple actions, lambda functions can simplify your code.
Every Function, Any Function:
Python’s any() and all() functions offer practical methods for verifying the truthiness of iterables. any() yields True when any of the iterable’s elements are true, and all() returns Only true if each and every element in the iterable is true. By enabling you to succinctly describe conditions involving several items, these methods help simplify and improve the expressiveness of conditional logic in your code.
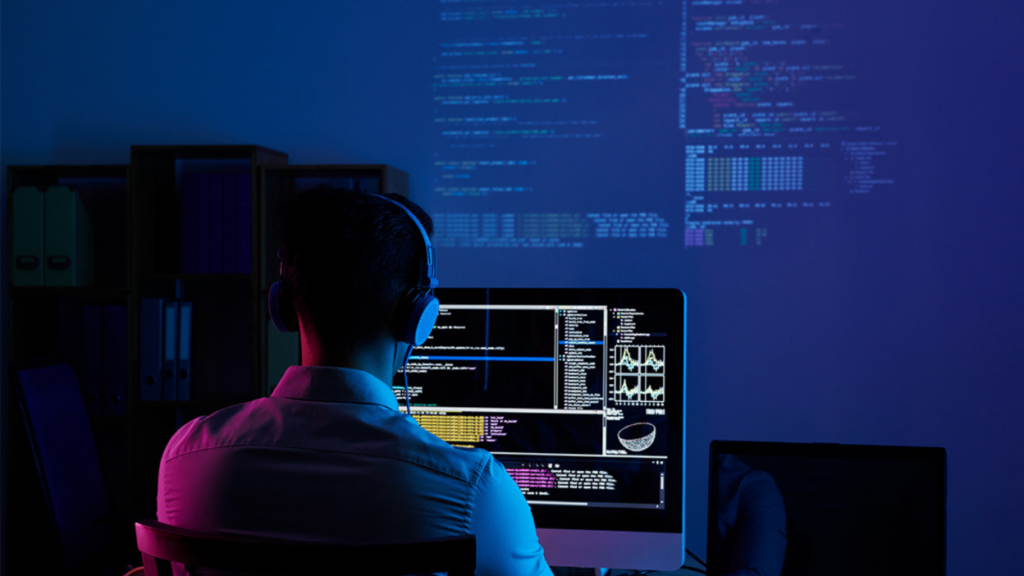
Enumerate Function:
To add counter values to Python iterable objects, use the enumerate() function. As you iterate over an iterable, it returns an enumerate object that yields pairs of index and value tuples. You may write more legible and succinct code for looping over sequences and maintaining track of the current index by using enumerate().
Zip Function:
Multiple iterables are combined into tuples with corresponding elements using the zip() function. It lets you create an iterator that returns tuples of elements from each iterable by allowing you to traverse over several sequences at once. Zip() comes particularly handy for transposing data structures or iterating over pairs of items in parallel. You may develop more expressive and effective code to handle data spanning many sequences by use zip().
Set Operations:
A number of built-in set operations, including union, intersection, difference, and symmetric difference, are available in Python’s set data structure. These procedures are very helpful for deduplication, membership checking, and filtering unique values since they may be used to handle sets of unique items in an efficient manner. You may develop more succinct and efficient code for handling collections of distinct elements by utilizing set operations.
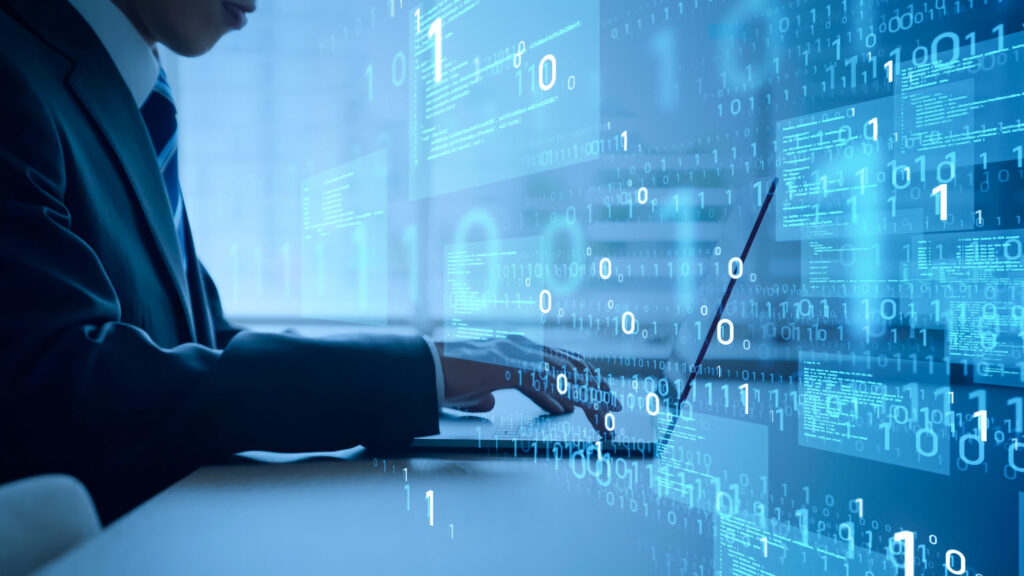
Dictionary Functions:
A wide range of built-in actions are available in Python dictionaries to facilitate common dictionary manipulations. Accessing keys and values, iterating over items, combining dictionaries, and other actions are among them. You can develop more expressive and effective code for activities like data transformation, lookup, and aggregation by utilizing dictionary operations.
Python Methods:
These 10 Python methods are strong tools that can help you make your code more readable and efficient. You may write more clear, expressive, and maintainable code and increase productivity and efficiency in your Python projects by implementing these techniques into your programming processes. Brilliko Institute of Multimedia provides knowledge about Python methods.